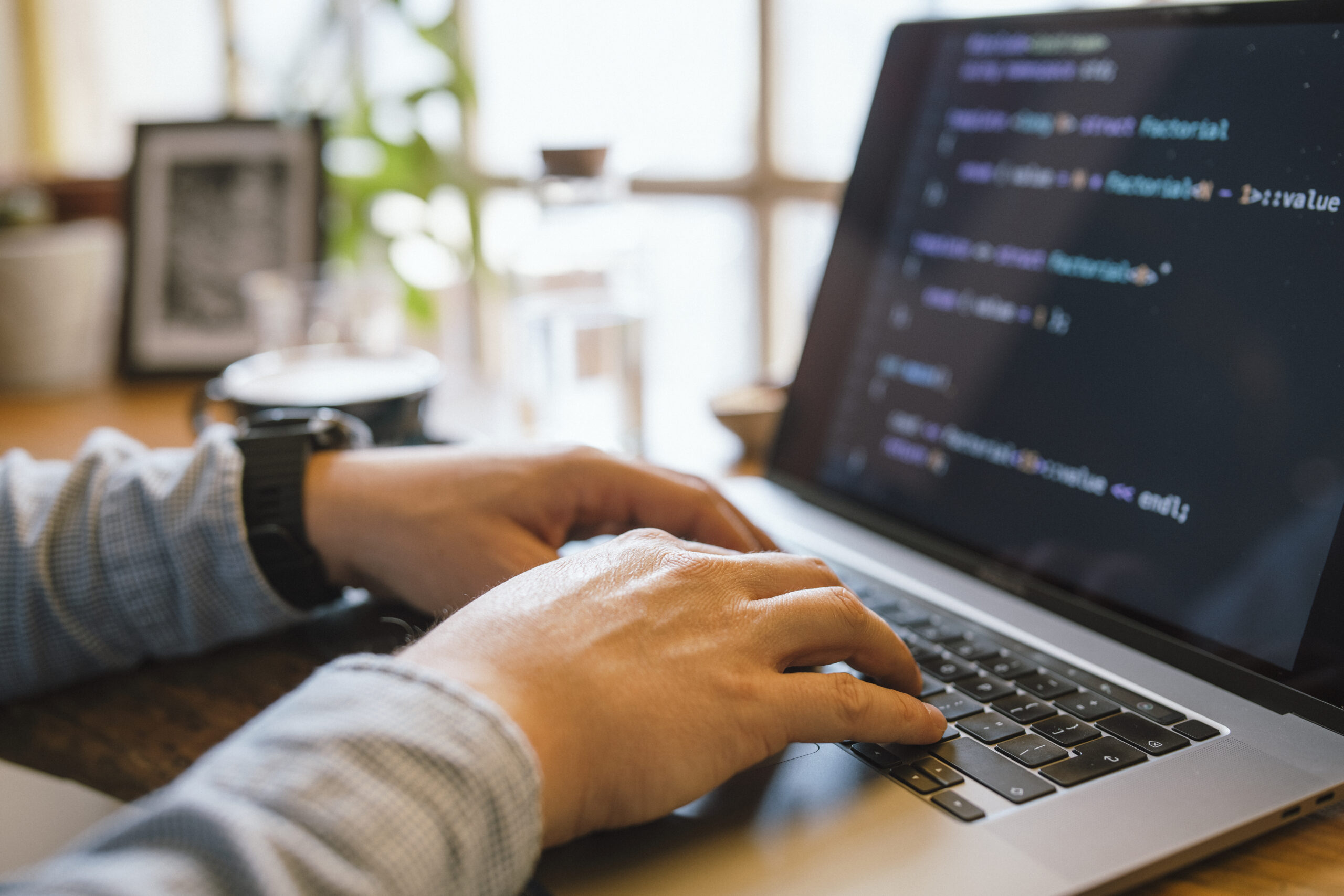
Debugging is Among the most essential — nevertheless generally neglected — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehending how and why items go Mistaken, and Mastering to Assume methodically to unravel complications effectively. No matter whether you're a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of irritation and radically help your efficiency. Here's various tactics to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Tools
Among the quickest methods developers can elevate their debugging skills is by mastering the applications they use everyday. Even though crafting code is just one Section of advancement, understanding how to connect with it correctly through execution is equally important. Modern-day growth environments come Geared up with impressive debugging abilities — but numerous builders only scratch the surface area of what these tools can perform.
Acquire, by way of example, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments enable you to set breakpoints, inspect the worth of variables at runtime, step by code line by line, and also modify code on the fly. When utilised accurately, they let you observe exactly how your code behaves for the duration of execution, that is priceless for tracking down elusive bugs.
Browser developer applications, like Chrome DevTools, are indispensable for entrance-end developers. They assist you to inspect the DOM, watch network requests, perspective actual-time general performance metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can switch frustrating UI troubles into workable tasks.
For backend or program-stage builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Finding out these resources could have a steeper Discovering curve but pays off when debugging overall performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, turn out to be at ease with version Manage techniques like Git to be aware of code record, find the exact minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools implies heading further than default configurations and shortcuts — it’s about acquiring an personal expertise in your development surroundings to make sure that when issues arise, you’re not lost in the dark. The better you know your resources, the greater time you may shell out fixing the actual difficulty as opposed to fumbling by means of the method.
Reproduce the challenge
The most essential — and sometimes ignored — actions in successful debugging is reproducing the situation. Ahead of jumping in to the code or making guesses, builders will need to make a regular surroundings or situation where the bug reliably seems. With no reproducibility, fixing a bug results in being a sport of chance, generally resulting in wasted time and fragile code improvements.
Step one in reproducing a problem is gathering just as much context as you can. Inquire thoughts like: What steps led to The difficulty? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it becomes to isolate the precise conditions underneath which the bug occurs.
When you finally’ve collected plenty of info, attempt to recreate the situation in your local natural environment. This could signify inputting exactly the same facts, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated exams that replicate the sting cases or condition transitions included. These tests not merely assistance expose the issue but also avoid regressions Down the road.
Occasionally, The problem can be atmosphere-distinct — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Using equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t just a stage — it’s a attitude. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more properly, test possible fixes safely, and communicate much more clearly together with your team or customers. It turns an abstract grievance into a concrete challenge — Which’s where by builders prosper.
Read through and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. Instead of seeing them as aggravating interruptions, developers should master to take care of error messages as direct communications within the system. They normally inform you just what happened, where by it took place, and at times even why it happened — if you know how to interpret them.
Get started by looking through the message carefully As well as in complete. Many builders, specially when below time pressure, look at the very first line and straight away start off creating assumptions. But further inside the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines — read through and comprehend them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can information your investigation and issue you toward the responsible code.
It’s also valuable to understand the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable patterns, and Mastering to recognize these can considerably speed up your debugging method.
Some faults are vague or generic, and in People conditions, it’s critical to look at the context by which the error transpired. Test related log entries, input values, and recent variations within the codebase.
Don’t forget about compiler or linter warnings both. These normally precede larger troubles and supply hints about opportunity bugs.
Ultimately, mistake messages are usually not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive tools in a developer’s debugging toolkit. When utilized successfully, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
A superb logging approach begins with realizing what to log and at what degree. Typical logging amounts consist of DEBUG, INFO, Alert, ERROR, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Information for common events (like thriving start-ups), Alert for probable troubles that don’t break the applying, Mistake for real problems, and Lethal in the event the process can’t proceed.
Steer clear of flooding your logs with too much or irrelevant facts. A lot of logging can obscure essential messages and decelerate your program. Target important situations, condition modifications, enter/output values, and significant choice details with your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re especially important in creation environments where by stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about equilibrium and clarity. Having a properly-assumed-out logging method, you could reduce the time it requires to identify issues, attain deeper visibility into your programs, and Increase the overall maintainability and reliability of your respective code.
Imagine Like a Detective
Debugging is not only a technological process—it is a method of investigation. To successfully recognize and deal with bugs, builders will have to method the method just like a detective resolving a secret. This state of mind aids break down intricate difficulties into workable sections and abide by clues logically to uncover the foundation result in.
Start off by collecting proof. Consider the indications of the trouble: mistake messages, incorrect output, or performance problems. Much like a detective surveys against the law scene, obtain just as much applicable information and facts as you are able to without having leaping to conclusions. Use logs, take a look at scenarios, and person reviews to piece with each other a clear picture of what’s happening.
Up coming, kind hypotheses. Request by yourself: What may be leading to this habits? Have any alterations just lately been manufactured on the codebase? Has this concern occurred before less than very similar conditions? The intention will be to slim down choices and identify opportunity culprits.
Then, exam your theories systematically. Endeavor to recreate the trouble inside a managed setting. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the results direct you closer to the reality.
Shell out close awareness to little aspects. Bugs typically hide while in the least envisioned spots—like a missing semicolon, an off-by-a person error, or simply a race issue. Be thorough and individual, resisting the urge to patch The difficulty without having absolutely knowing it. Non permanent fixes could disguise the real trouble, only for it to resurface later on.
Last of all, preserve notes on click here Anything you attempted and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for potential challenges and assist Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical capabilities, tactic issues methodically, and grow to be more practical at uncovering concealed issues in sophisticated programs.
Generate Tests
Creating exams is among the simplest methods to boost your debugging capabilities and Over-all development efficiency. Tests not just aid catch bugs early but will also function a security Web that offers you assurance when creating adjustments in your codebase. A properly-examined application is simpler to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device assessments, which target particular person features or modules. These modest, isolated assessments can immediately expose no matter if a certain piece of logic is Functioning as anticipated. When a test fails, you immediately know where by to glance, appreciably lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Following, combine integration tests and end-to-conclusion exams into your workflow. These assist ensure that many areas of your application do the job collectively smoothly. They’re significantly useful for catching bugs that come about in sophisticated systems with many elements or products and services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and less than what problems.
Producing tests also forces you to definitely Believe critically regarding your code. To test a attribute properly, you require to know its inputs, predicted outputs, and edge instances. This standard of comprehending naturally qualified prospects to raised code construction and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails continuously, you are able to focus on fixing the bug and look at your exam pass when The problem is fixed. This approach makes sure that the exact same bug doesn’t return Down the road.
In brief, composing assessments turns debugging from the aggravating guessing video game right into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult situation, it’s quick to be immersed in the problem—watching your display screen for hrs, seeking Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower aggravation, and often see the issue from the new standpoint.
If you're much too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code that you just wrote just several hours before. With this condition, your brain turns into significantly less effective at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious function during the qualifications.
Breaks also aid stop burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally trapped, is not simply unproductive but additionally draining. Stepping absent lets you return with renewed Vitality and a clearer way of thinking. You could abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may come to feel counterintuitive, especially beneath tight deadlines, nonetheless it actually brings about quicker and simpler debugging in the long run.
In a nutshell, using breaks will not be a sign of weak point—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is part of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each can instruct you some thing useful in case you go to the trouble to reflect and analyze what went Incorrect.
Start off by inquiring yourself a couple of essential issues as soon as the bug is fixed: What brought on it? Why did it go unnoticed? Could it are already caught previously with better practices like unit testing, code reviews, or logging? The answers frequently reveal blind spots in your workflow or understanding and assist you to Develop stronger coding habits moving ahead.
Documenting bugs can even be an outstanding habit. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see designs—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing That which you've realized from a bug with your friends is often Specially potent. Whether or not it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a more powerful Studying society.
Extra importantly, viewing bugs as lessons shifts your mindset from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital parts of your growth journey. In the end, a lot of the greatest builders usually are not those who create great code, but those that consistently discover from their faults.
In the end, each bug you correct provides a fresh layer on your skill established. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and persistence — even so the payoff is large. It can make you a far more efficient, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.